We have written extensively on the types of image annotation on our blog, and now we would like to shift our focus to one of the most important applications of computer vision: object detection. Computer vision aims to teach machines how to “see” and make sense of visual information. Object detection is commonly the first step in identifying and locating objects within images or video frames.
This technique is crucial because, unlike simple image classification—which assigns one or more labels to an entire image—object detection pinpoints the specific location of each object, often through bounding boxes, while also labeling these objects and attaching related metadata.
In recent years, object detection has made a substantial impact across multiple industries, including autonomous driving, retail, security, healthcare, and robotics. The combination of computer vision with bounding boxes is widely recognized because it provides a straightforward, easy-to-understand representation for the general public.
Car Detection with Bounding Box | Unitlab Annotate
By the end of this post, you will learn:
- Essentials of object detection
- How object detection works
- Real-world applications
- Demo object detection project
- Common challenges and suggested solutions
What is Object Detection?
Object detection is a branch of computer vision focused on discovering objects of interest in an image or video and marking their positions with bounding boxes, accompanied by labels. It merges two core tasks of computer vision:
- Classification – Determining which objects are present in the image.
- Localization – Pinpointing the precise coordinates of those objects.
As described in our previous article on bounding boxes, object detection depends on coordinates. For instance, a detected object might look like this:
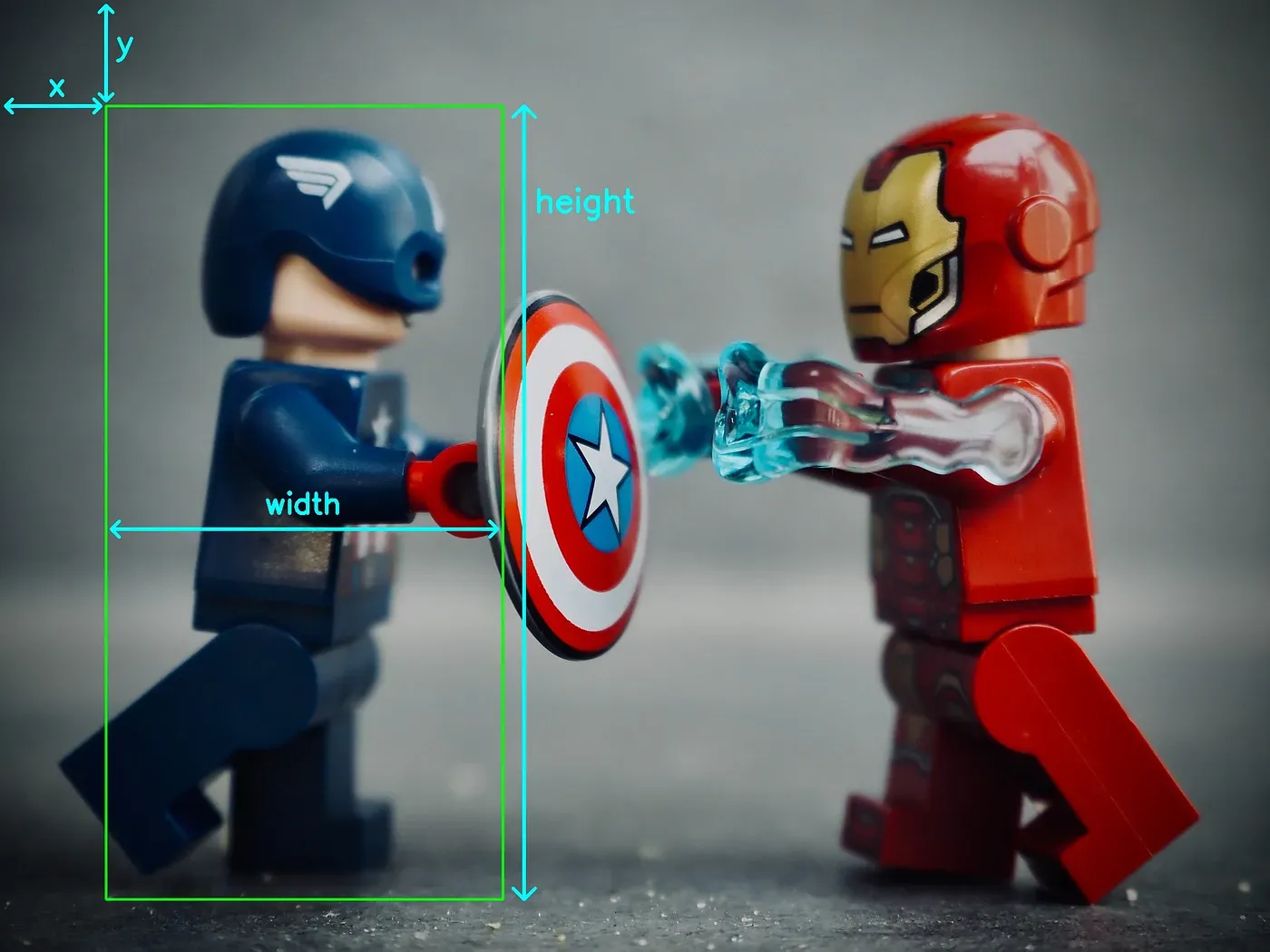
{
'class': 'Person',
'coordinates': {
'x': 10,
'y': 10,
'width': 150,
'length': 300
},
'confidence_score': 0.93,
}
A machine would interpret this information as: “The area within these coordinates belongs to an instance of a person.” Earlier iterations of object detection were performed by human annotators, but modern methods rely predominantly on AI/ML models.
These models are trained on labeled datasets such as COCO, where each image or frame is annotated with object categories, bounding boxes, and other relevant details. By learning from large amounts of labeled data, these algorithms can recognize objects in new, unseen images by extracting and comparing features to what they have already learned.
Some of the most notable detection architectures include:
- YOLO (You Only Look Once) – A series of efficient and fast detectors, including the YOLOv8 version we will use in our demo.
- Faster R-CNN (Region-based Convolutional Neural Network) – A two-stage detector that is valued for its accuracy.
Applications of Object Detection
Because object detection is a foundational method for enabling machines to interpret their visual surroundings, it appears in a wide range of applications, including the following:
-
Autonomous Vehicles
- Real-time identification of pedestrians, traffic signs, and other vehicles to help maintain safe navigation in complex environments.
- Enhancement of advanced driver-assistance systems (ADAS) with real-time hazard detection.
-
- Automated detection and tracking of product stock levels on store shelves.
- Real-time monitoring of inventory for more efficient audits.
-
Security & Surveillance
- Identifying and detecting unauthorized access in restricted or sensitive areas.
- Integrating facial recognition tools to monitor public spaces.
-
Agriculture & Farming
- Detecting pests or plant diseases to streamline interventions.
- Observing livestock populations and tracking their movement patterns.
Demo Object Detection Project
For a straightforward example, we will build a simple object detection model using YOLOv8 that draws bounding boxes around recognized objects in an image. It just takes an image as an input and draws bounding boxes around the objects that it can identify as an output. You can find all the relevant code and installation instructions in our GitHub repository.
Below are the steps to implement a basic object detection demo using Python and OpenCV:
Step 1:
pip install ultralytics opencv-python numpy
Here, we import our libraries and load the YOLOv8 model.
Step 2:
# main.py
import cv2
from ultralytics import YOLO
# Load YOLOv8 model
yolo_model = YOLO("yolov8n.pt")
# Load an image
image_path = "test.jpg"
image = cv2.imread(image_path)
We import the necessary models into our code in order to perform object detection. We will use the YOLOv8n as our model. And finally read the test image:
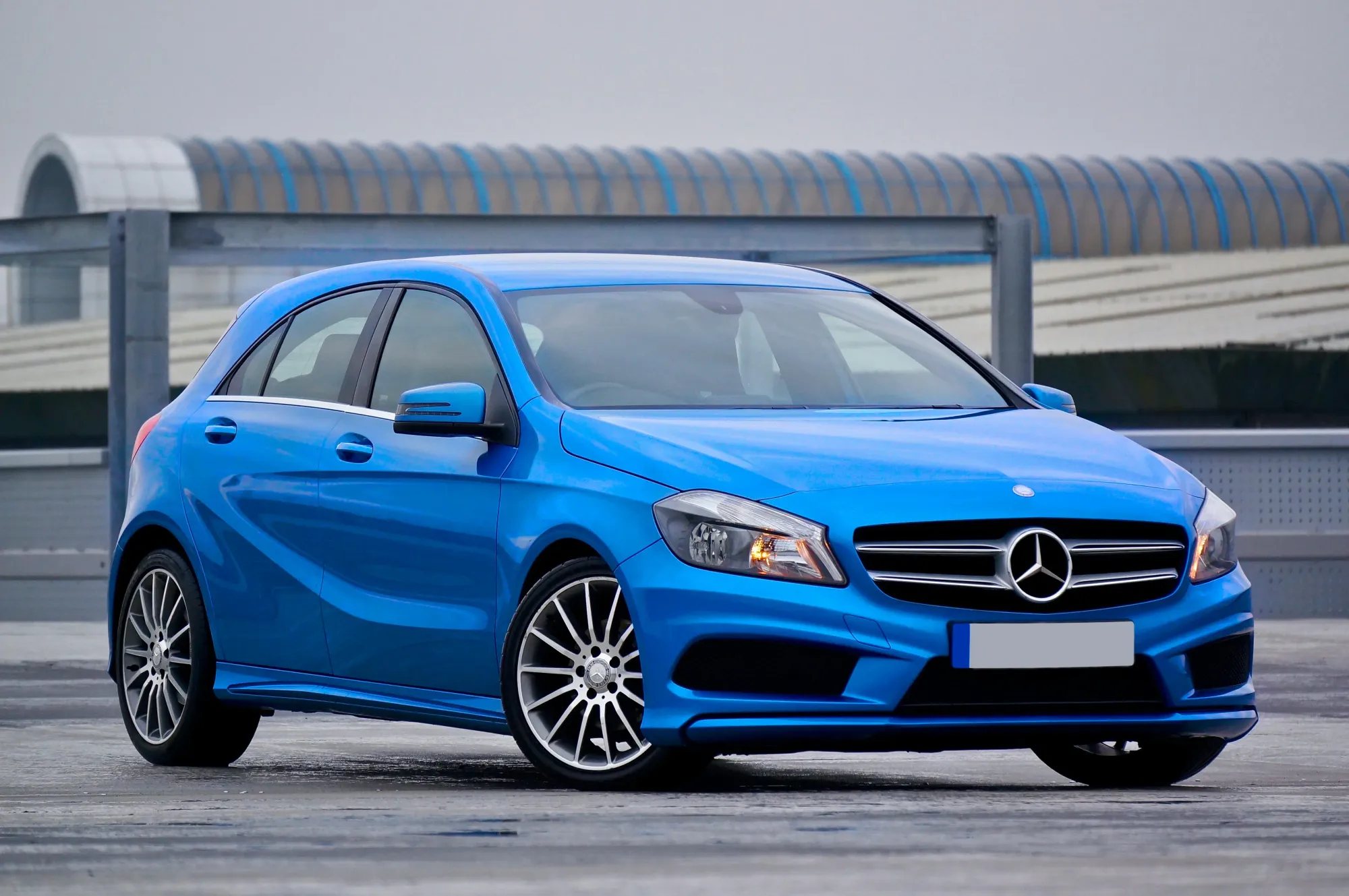
Step 3:
# Perform detection
results = yolo_model(image)
# Draw bounding boxes and display results
for result in results:
for box in result.boxes:
x1, y1, x2, y2 = map(int, box.xyxy[0])
label = result.names[int(box.cls[0].item())]
confidence = box.conf[0]
# Draw bounding box
cv2.rectangle(image, (x1, y1), (x2, y2), (0, 0, 255), 5)
cv2.putText(
image,
f"{label}: {confidence:.2f}",
(x1, y1 - 10),
cv2.FONT_HERSHEY_SIMPLEX,
1.2,
(0, 0, 255),
4,
)
cv2.imshow("Detection", image)
cv2.waitKey(0)
In this step, first we load the image and 'read' it using the OpenCV. Now, the output is a bounding box drawn around the car:
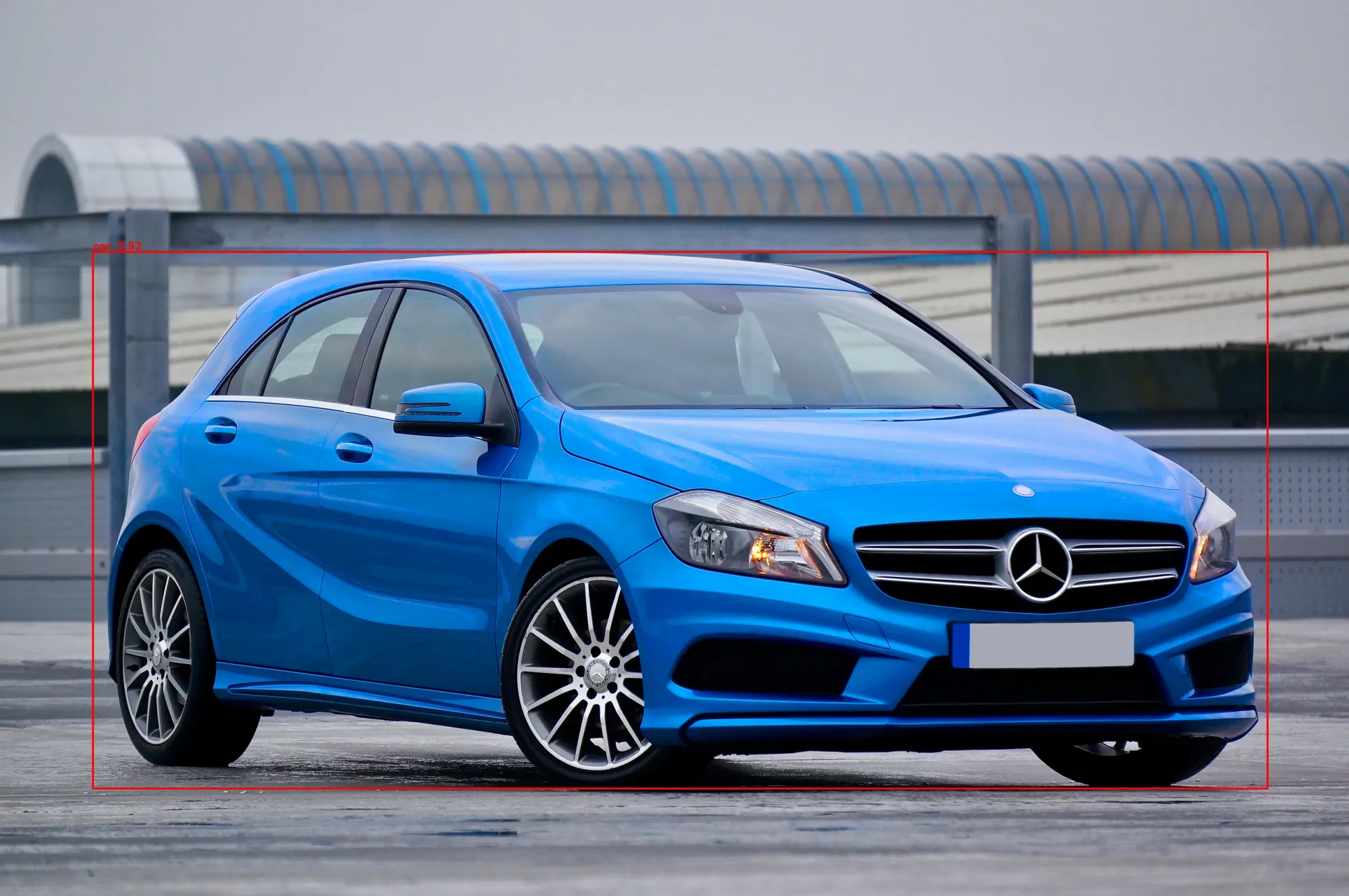
Actually, the object detection part is absurdly easy to implement:
results = yolo_model(image)
The model takes care of the hard work, returning the labeled bounding boxes and confidence scores. While this simple example is a nice demonstration, it does not address a concrete practical task. Real-world projects require comprehensive datasets and careful data annotation. If you need more information on the significance of annotation platforms and how to select one, you can explore more on this post:
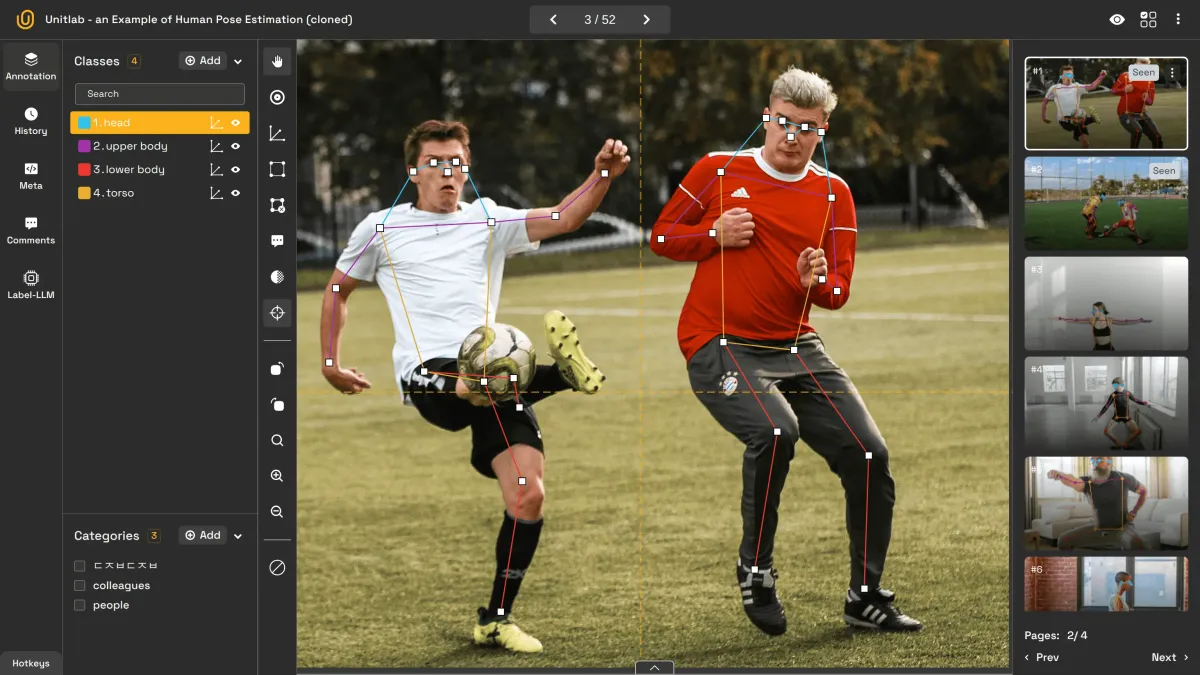
11 Factors in Choosing Image Annotation Tools | Unitlab Annotate
Challenges and Solutions
Like any piece of technology worth building, object detection comes with its own set of challenges and solutions:
-
Small Object Detection
- Problem: Models often struggle with accurately identifying very small objects.
- Solution: Use higher-resolution imagery and algorithms designed for smaller targets (such as Faster R-CNN).
-
Occlusions & Overlapping Objects
- Problem: Occluded and overlapping objects reduce detection accuracy.
- Solution: Combine object detection with instance segmentation (e.g., Mask R-CNN), and ensure all occluded objects are labeled properly; otherwise, you risk this:
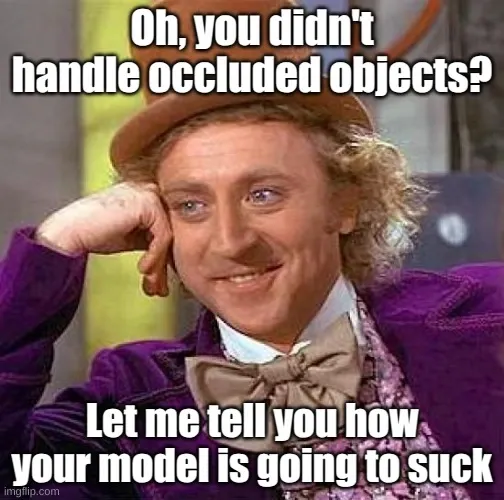
-
Real-time Performance
- Problem: Achieving real-time inference requires substantial computing power, specialized hardware, and robust infrastructure.
- Solution: Select lighter models like YOLOv8-tiny for low-latency use cases, and optimize your deployment environment.
-
Data Annotation and Quality
- Problem: Poorly annotated datasets inevitably lead to ineffective models. (garbage in, garbage out).
- Solution: Introduce a human-in-the-loop approach or labeling and diversify your training data to improve generalization.
Conclusion
Object detection remains one of the core capabilities within the broader field of computer vision, and it continues to evolve. Its capacity to identify objects in images and videos benefits countless industries, from boosting safety in autonomous vehicles to supporting medical diagnostics and optimizing retail operations.
In this overview, we discussed how object detection works, examined notable use cases, reviewed common pitfalls and remedies, and walked through a basic implementation of YOLOv8. As a vital facet of computer vision, object detection will undoubtedly continue to transform how machines interpret and engage with visual information.
Explore More
- Guide to Pixel-perfect Image Labeling
- Importance of Clear Guidelines in Image Labeling
- 5 Tips for Auto Labeling
References
1. James Gallagher. (Nov 26, 2024). What is Object Detection? The Ultimate Guide [2025]. Roboflow Blog: https://blog.roboflow.com/object-detection/